How to run the python code in the Flutter app?
Running any language code in the background of the app is a bit tough process, to make it easy in the flutter there is one package called Starflut.
In this blog, we discuss how to run python files in the back end.
Step 1:
Create a Flutter new project: flutter create
Step 2:
Add starflut package in pubspec.yaml as starflut:
Step 3:
Click the following link to download the required file. https://drive.google.com/file/d/1GdiQqoQB8jlwafW34URahf4SpWh3-Pcx/view?usp=sharing
After downloading extract it and copy the assets and jniLibs folder and paste it in android>app>src>main. And copy the starfiles folder then paste it in the flutter source folder
Step 4:
Include the starfiles folder in pubspec.yaml in the assets group.
assets:
- starfiles/
Step 5:
Rewrite the main file with the following code.
import 'package:flutter/material.dart';
import 'dart:async';
import 'package:flutter/services.dart';
import 'package:starflut/starflut.dart';
void main() => runApp(new MyApp());
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => new _MyAppState();
}
class _MyAppState extends State<MyApp> {
String _platformVersion = 'Unknown';
@override
void initState() {
super.initState();
initPlatformState();
}
// Platform messages are asynchronous, so we initialize in an async method.
Future<void> initPlatformState() async {
String platformVersion;
// Platform messages may fail, so we use a try/catch PlatformException.
try {
StarCoreFactory starcore = await Starflut.getFactory();
StarServiceClass Service = await starcore.initSimple("test", "123", 0, 0, []);
await starcore
.regMsgCallBackP((int serviceGroupID, int uMsg, Object wParam, Object lParam) async {
print("$serviceGroupID $uMsg $wParam $lParam");
return null;
});
StarSrvGroupClass SrvGroup = await Service["_ServiceGroup"];
/*---script python--*/
bool isAndroid = await Starflut.isAndroid();
if (isAndroid == true) {
await Starflut.copyFileFromAssets(
"testcallback.py", "flutter_assets/starfiles", "flutter_assets/starfiles");
await Starflut.copyFileFromAssets(
"testpy.py", "flutter_assets/starfiles", "flutter_assets/starfiles");
await Starflut.copyFileFromAssets(
"python3.6.zip", "flutter_assets/starfiles", null); //desRelatePath must be null
await Starflut.copyFileFromAssets("zlib.cpython-36m.so", null, null);
await Starflut.copyFileFromAssets("unicodedata.cpython-36m.so", null, null);
await Starflut.loadLibrary("libpython3.6m.so");
}
String docPath = await Starflut.getDocumentPath();
print("docPath = $docPath");
String resPath = await Starflut.getResourcePath();
print("resPath = $resPath");
dynamic rr1 = await SrvGroup.initRaw("python36", Service);
print("initRaw = $rr1");
var Result = await SrvGroup.loadRawModule(
"python", "", resPath + "/flutter_assets/starfiles/" + "testpy.py", false);
print("loadRawModule = $Result");
dynamic python = await Service.importRawContext("python", "", false, "");
print("python = " + await python.getString());
StarObjectClass retobj = await python.call("tt", ["hello ", "world"]);
print(await retobj[0]);
print(await retobj[1]);
print(await python["g1"]);
StarObjectClass yy = await python.call("yy", ["hello ", "world", 123]);
print(await yy.call("__len__", []));
StarObjectClass multiply = await Service.importRawContext("python", "Multiply", true, "");
StarObjectClass multiply_inst = await multiply.newObject(["", "", 33, 44]);
print(await multiply_inst.getString());
print(await multiply_inst.call("multiply", [11, 22]));
await SrvGroup.clearService();
await starcore.moduleExit();
platformVersion = 'Python 3.6';
} on PlatformException catch (e) {
print("{$e.message}");
platformVersion = 'Failed to get platform version.';
}
// If the widget was removed from the tree while the asynchronous platform
// message was in flight, we want to discard the reply rather than calling
// setState to update our non-existent appearance.
if (!mounted) return;
setState(() {
_platformVersion = platformVersion;
});
}
@override
Widget build(BuildContext context) {
return new MaterialApp(
home: new Scaffold(
appBar: new AppBar(
title: const Text('Plugin example app'),
),
body: new Center(
child: new Text('Running on: $_platformVersion\n'),
),
),
);
}
}
Step 6:
Run and build the flutter app, and you will see python code debug and output in the console.
Code Explanation:
Python
In this program, we wrote a python file with 1 variable, 1 class, and 2 definitions.
g1 = 123
G1 is one global variable with value 123
def tt(a,b) :
print(a,b)
return 666,777
tt is one function that gets 2 parameters. In this, you can notice that 1 print statement and 1 return statement is there. Actually print statement just print data in the console, but the return statement return data to flutter.
def yy(a,b,z) :
print(a,b,z)
return {'jack': 4098, 'sape': 4139}
This is the same as the previous function but this returns us JSON value.
class Multiply :
def __init__(self,x,y) :
self.a = x
self.b = y
def multiply(self,a,b):
print("multiply....",self,a,b)
return a * b
This is class with one multiply which returns the multiplied value of the given parameter.
Flutter:
In this app, we just run those python files in the background and print the output in the console and dummy UI.
@override
Widget build(BuildContext context) {
return new MaterialApp(
home: new Scaffold(
appBar: new AppBar(
title: const Text('Plugin example app'),
),
body: new Center(
child: new Text('Running on: $_platformVersion\n'),
),
),
);
}
Simple UI which print the platform version, here it will print python3.6
@override
void initState() {
super.initState();
initPlatformState();
}
Init function contains the new function which has all the setup needed to run python code in it.
Now we look into what that function contains.
StarCoreFactory starcore = await Starflut.getFactory();
StarServiceClass Service = await starcore.initSimple("test", "123", 0, 0, []);
await starcore.regMsgCallBackP(
(int serviceGroupID, int uMsg, Object wParam, Object lParam) async{
print("$serviceGroupID $uMsg $wParam $lParam");
return null;
});
StarSrvGroupClass SrvGroup = await Service["_ServiceGroup"];
/*---script python--*/
bool isAndroid = await Starflut.isAndroid();
if( isAndroid == true ){
await Starflut.copyFileFromAssets("testcallback.py", "flutter_assets/starfiles","flutter_assets/starfiles");
await Starflut.copyFileFromAssets("testpy.py", "flutter_assets/starfiles","flutter_assets/starfiles");
await Starflut.copyFileFromAssets("python3.6.zip", "flutter_assets/starfiles",null); //desRelatePath must be null
await Starflut.copyFileFromAssets("zlib.cpython-36m.so", null,null);
await Starflut.copyFileFromAssets("unicodedata.cpython-36m.so", null,null);
await Starflut.loadLibrary("libpython3.6m.so");
}
String docPath = await Starflut.getDocumentPath();
print("docPath = $docPath");
String resPath = await Starflut.getResourcePath();
print("resPath = $resPath");
dynamic rr1 = await SrvGroup.initRaw("python36", Service);
print("initRaw = $rr1");
These are the init step, to copy the necessary assets to run the python file.
var Result = await SrvGroup.loadRawModule("python", "", resPath + "/flutter_assets/starfiles/" + "testpy.py", false);
print("loadRawModule = $Result");
Load the testpy.py file and check whether it loaded or not.
dynamic python = await Service.importRawContext("python", "", false, "");
Create object python which is used to call python definition and variables.
print(await python["g1"]);
This will get the variable value from the python and use it in a flutter.
StarObjectClass retobj = await python.call("tt", ["hello ", "world"]);
print(await retobj[0]);
print(await retobj[1]);
// Output:
// I/<string>,2(25176): hello world
// I/flutter (25176): 666
// I/flutter (25176): 777
In this way, you can call a function in flutter and save it to a variable or object, and later you can use it for further purpose.
StarObjectClass multiply = await Service.importRawContext("python", "Multiply", true, "");
StarObjectClass multiply_inst = await multiply.newObject(["", "", 33, 44]);
print(await multiply_inst.getString());
print(await multiply_inst.call("multiply", [11, 22]));
In the same way, you can make an object for class and call the function inside the class.
await SrvGroup.clearService();
await starcore.moduleExit();
Clear the service once the purpose is over.
In this blog, we discuss how to run a python files inside flutter and understand how to call functions.
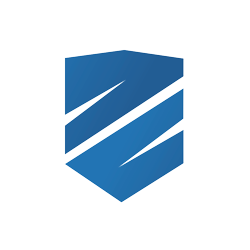