Learn React Basics in 10 mins
Difficulty : Beginner to Intemediate
React Approach : Functional Component Based
Prerequiste :
- Visual Studio Code / Your favourite IDE
- NodeJs
- npm / yarn
- Google Chrome
- Git knowledge
Before getting into React you should have good skills on JavaScript especially on ES6 ( ES2015 ) or further patch versions. If you know JavaScript well do have a check here : Refresh JS on Mozilla Developer Networks if you dont know much of JavaScript following links will be useful for you
Once you have skimmed through all the above links or have a good knowledge of the concepts of JS, just make sure have good understanding of the following
Arrow Functions
Introduced along with ES6 is a wonderful replacement of the old regular function. Arrow functions are used in scripts whenever there is a callback or you need to isolate the this variable from the methods.
// ES5 or earlier
function test(){
console.log("Hello");
}
// ES6 Arrow Funciton
const test = () =>{
console.log("World");
}
Let & Const
ES6 releases also brought the concept of let and const. Two new ways of declaring a variable along with the existing var. These three declaration types are majorly differentiated with there life-cycle scope and along with the rules they are associated with.
// var
var test = "hello";
printTest:()=>{
console.log(test); // prints hello | var scope based on where its initalized
test = "world";
console.log(test); // prints world | var is a global scope and could be modified
}
// let
let test = "hello";
printTest:()=>{
console.log(test); // prints undefined | let is block scope (i.e) lives between {...}
let test = "world";
console.log(test); // prints world | let is bloack scope and works with in the block
}
// const
const test = "hello";
printTest:()=>{
console.log(test); // prints hello | const scope based on where its initalized
test = "world";
console.log(test); // throws error | const cannot be modified
}
Callback Methods
Callback as the name suggests are methods which are called after a certain operations is completed or duration. Callback methods are the pillars for making the JavaScript from Syncchronouse to Asynchronous. A method is determined as callback by following validations
- When a Function is passed as a variable
- When a Function is passed as a parameter
total:(subtotal,name)=>{
console.log(`Hi ! ${name} , cost of website development is : ${subtotal}`);
}
calculation:(design,development,customer,callback)=>{
let subtotal = design + development;
callback(subtotal,customer); // callback is not a keyword, just a variable triggers the method
}
calculation("creative","innovation","you",total); // function name is passed as a variable
Promise
Callback may sound simpler for small scale use-cases, but in a larger picture, callback leads to confusion so at that times or based on use-cases we prefer promise. Promise as name suggests.
- If Smazee promises to deliver client a wonderful project, client will definitely be awaiting for the end result to verify it's wonderful or not ||
resolve or reject.
- Similarly Smazee provides the client with frequent updates on the progress through smazee agile methodology work culture with review and meetings ||
pending status or rejected or settled or fulfilled
In general, promise is an amalgamation of multiple callbacks as one, provided with a simpler way of processing the callback through its prop methods
- resolve
- reject
and promise are generally wrapped by then ( for both reject or resolve cases ) else reject on catch block
const total = 40;
cosnt promise = new Promise((res,rej)=>{ // res rej are not keywords, they are just method name as params
let sub = 20+20;
if(sub === total){
res("equal"); // calls a specific method if its true
}else{
rej("not equal"); // calls a specific method if its false
}
}).then((res)=>{
console.log(res); // prints only when the response is resolved
}).catch((err)=>{
console.log(err); // prints only when the resposne is rejected
});
Async & Await
Working on Promises are quite schematic and easy to understand, but while trying nested loops, many time the API may have to get a request so at that times aync function comes handy and converts the program to synchronous. Both of these are keywords which compiles the methods in a parallel way. Few rules before you go into this deep
- await should be used only inside async function
- async function always returns a value so bound the method with then and catch to get the returned value
- async keyword should be present while declaring a function
async function test(){
...loop
...methods
...api calls
return true
}
test().then((res)=> // res is just a parameter to obtain the return value
{
cosnole.log(res); // true gets returned
});
// await
function test(){
const pro = new promise((r,x)=>{
if(10>5){ r("true");}else{x("false");}
}).then((res){console.log(res);}).catch((err){console.log(err);});
}
async function testmain(){
console.log("init first");
let wait = await test(); // during await it pauses the particular block alone
console.log("after await");
}
Array Methods
Array methods are functions attached along with the array to perform an operation over the elements on the array. There are around 10+ Methods ( Sort, Iteration, Operation ) but here we are concentrating on few of the important. But do check out the following links to learn more in detail
foreach
By the name its self explanatory that the following method would run an operation for each element in the array an iteration. By default the foreach as three param namely
- value - item value
- index - item index
- array - all items
var arr = [1,3,4,5];
arr.foreach(test());
test:(value)=>{
console.log(value++); // 2,4,5,6
}
Map
Partially similar to the foreach method, but performs the same operation and returns the end result as separate array : end result.
var arr = [1,2,3,4];
var arr2 = arr.map(test());
test:(value)=>{
return value++;
}
// arr : 1,2,3,4
// arr2: 2.3.4.5
I hope you might have got confused, where is the react part as almost half of the blog covered JavaScript. Yes! you are right, React is quite quick to learn once we make the JavaScript strong or have a good understanding of the same.
Infact in further few scroll we will learn few concepts, which may sound similar to the one earlier we have learnt with
- React Hooks - Works on Callback techniques
- Components or React Methods - Works on Arrow Funcitons
- Declaration of React library elements - Works on let & const
Now lets dive deep into the world of React
Q1. What is React ?
React is a JavaScript library developed by Facebook. React since its release on the year 2015, it evolved to be the most used language with a wonderful community developing resources. In general React is the View on any MVC Framework.
Q2. Then what's React Native ?
Yup its a question that confuses you often, but React Native is entirely different but uses React as base with Native elements. Will be explained detailed in the next blog!
Q3. React Architecture ?
React architecture includes the power of Document Object Model on any given browser. Architecture involves many pillars which would be discussed later, but in general React uses a virtual DOM concept, which makes it UI First library
- Rendering are powerful and memory saving
- Only the modifies content updates
- Not damaging the State information
Q4. React Pillars
These are the four pillars, of React mainly, on which we will look deeper down on this blog
- Component
- Props
- State
- JSX
Component
Components are building blocks of React. They are nothing but a simple (.js) file on which our functions or the business logic are written. With the help of node we get to import modules from react-js npm. Components usually return rendering elements.Component could be called inside another component.
Props
Props are the data that are passed on to the component externally. Props are read-only.Props data are Scope of the component alone. Passed as an object to the Component.
State
State are similar to the variables with unique properties / rules to begin with. In general state are placeholder for storing a certain set of information which could be later updated over the application run time. Given the help of virtual DOM, only the state elements gets updated instead of the whole component itself. States are most important in handling data and React have two approaches
- Class Component
- Functional Component
State methods, properties differ according to the approach you prefer to develop with.This blog will showcase examples of Funcitonal COmponent
JSX
JSX stands for Java Script Extendable Markup Language. JSX adds power to the React in handling UI as well the Javascript data on single (.js) file without having two separate files. JSX are allowed to have single parent in React.
Enough of theory on react, lets get on the building part. Make sure you have your system setup with the proper software mentioned above
Let's Hack
Open your terminal on VSCode on a folder where you want to save the project or keep the files and type the following
npx create-react-app my-app
- my-app here is the app name you want to keep
- create-react induces the node to download the necessary modules for react
- npx - is a npm package runner, provides to install dependencies directly to local folder
Later type the following
cd my-app
yarn start
- cd - change directory to the app folder
- starts the react server on
http://localhost:3000/
After couple minutes, you may see your app running on the localhost server!
File Structure of a React Project
node_modules
- In general for every node project you will be having this folder, quite common and contians all the dependcies modules
- Add this directory to gitignore before you push the code
public
- though with help of JSX we solve the issue of having two files for the UI on html and logic on js, react maintains a drecotry public which at last compiles the scripts to a browser readable from
- its advised initally to not edit or modify here
package.json
- the config file of our project
- contains all the dev dependices and app related scripts
src
-
this is the mother of our project which contains most of the files we will be working on
index.js
-
entry point of the project
-
In other words this is the only file will be executed till the end of the project only by switching through the component under the React.DOM.render
// index.js import React from 'react'; // importing a module exported from node_modules import ReactDOM from 'react-dom'; import './index.css'; // importing files in directory import App from './App'; // importing a component import * as serviceWorker from './serviceWorker'; // offline support ReactDOM.render( // Renders the app <React.StrictMode> // root element <App /> // component is called here </React.StrictMode>, document.getElementById('root') // attached to the root element seen on public/index.html );
at the end it's a single file which is rendered with components alone re-rendered with page remained stable instead of refresh
app.js
-
the component file
// App.js import React from 'react'; // imports import logo from './logo.svg'; import Search from './Components/search'; import './App.css'; function App() { // Functional Component with Name return ( // renders the block whenever the app component is called <div className="App"> // JSX Elements ( single parent ) <h1>Hey !</h1> // UI Elements </div> ); } export default App; // Funcitonal component export
-
each component should be exported with
export default component-name;
-
React Hooks and State
As this blog we are using functional component examples, state in functional component are normally dealt with Hooks
- Hooks are generally the concept of appending a new state using a callback methods as hooks
useState() useEffect() useContext() useCallback()
are few of the hooks example- working of hooks are quite similar to a callback
- We can also create custom Hooks
import React from "react";
import {useState} from "react";
function hooksSample(){
const [number,setNumber] = useState(0);
// 0 - initalized state or number of the hook
// number - it's read-only with state value of 0
// setNumber - it's the method that modifies state
// hooks should be initailized inside the component function
hookChange:()=>{
console.log(number); // 0
setNumber(2); // callback methods gets called and the state is changed
console.log(number); // 2
}
return(
<div>
<button onClick={hookChange}/> // JSX elements should always be enclosed
</div>
);
}
export default hooksSample;
React props
// mainfile | App.js
import React from "react";
import 'Test' from './Test'; // Component is called
function App(){
return(
<div>
<Test header="Hey Hi!"/>
</div>
);
}
export default App;
// component file | Test.js
import React from "react";
function Test(props){ // props are the vairable for the params of component
return(
<div>
<h1>{props.header}</h1> // calling the props
</div>
);
}
export default Test;
// Output would be "Hey Hi" in header tag!
Next >
So far, you have seen most of the beginner level concepts in hopefully a better way, following are the few concepts we will be covering in Next Series
- Redux Concepts
- Custom Hooks
- Testing in React
- UI Management Library : StoryBook
Thanks!
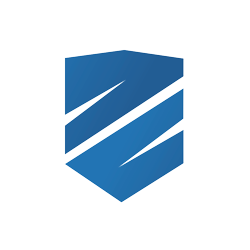